Adding SDK to App
Overview
Supports Android Mobile, Android TV, and Fire TV applications.
The Android SDK can be implemented 2 different ways:
- Add SDK using Maven
- Import
.aar
package
Dependencies
The OneTrust SDK uses the following dependencies. Make sure to include them or exclude them based on your current project. Not having these will cause runtime exceptions.
// mandatory
implementation 'androidx.appcompat:appcompat:1.6.1'
implementation 'androidx.constraintlayout:constraintlayout:2.1.4'
implementation 'com.google.android.material:material:1.11.0'
implementation 'androidx.work:work-runtime:2.9.0'
implementation 'androidx.browser:browser:1.7.0'
implementation 'com.github.bumptech.glide:glide:4.10.0'
implementation 'androidx.lifecycle:lifecycle-viewmodel-ktx:2.7.0'
implementation 'androidx.fragment:fragment-ktx:1.6.2'
implementation "org.jetbrains.kotlin:kotlin-stdlib:1.7.10"
implementation 'com.squareup.retrofit2:converter-scalars:2.8.1'
// optional: Only when the SDK needs to measure Google Ad Id Opt In/Outs
implementation 'com.google.android.gms:play-services-ads-identifier:18.0.1'
// optional for QR code display on TV UI
implementation 'com.google.zxing:core:3.3.0'
// for cmp api implementations
implementation "org.jetbrains.kotlinx:kotlinx-coroutines-core:1.6.4"
implementation "org.jetbrains.kotlinx:kotlinx-coroutines-android:1.6.4"
Add the following code snippet to the 'android()' section of the app's gradle file
buildFeatures {
viewBinding true
}
Known Issues with Gradle Version 8+
There is a known issue with gradle version 8+ where the application is getting a build error with R8 with the OneTrust SDK.
Solution 1: Add the optional dependencies below in the app's build.gradle file
implementation ("com.google.android.gms:play-services-ads-identifier:18.0.1")
implementation ("com.google.zxing:core:3.3.0")
Solution 2: In app's proguard-rules.pro file, add the rules below:
# Ignoring implementation ("com.google.zxing:core:3.3.0") dependencies.
-dontwarn com.google.zxing.BarcodeFormat
-dontwarn com.google.zxing.EncodeHintType
-dontwarn com.google.zxing.MultiFormatWriter
-dontwarn com.google.zxing.common.BitMatrix
# Ignoring implementation ("com.google.android.gms:play-services-ads-identifier:18.0.1") dependencies
-dontwarn com.google.android.gms.ads.identifier.AdvertisingIdClient$Info
-dontwarn com.google.android.gms.ads.identifier.AdvertisingIdClient
Known Runtime Issue with Gradle Version 8+ if isMinifyEnabled = true
isMinifyEnabled = true
With Gradle version 8+, if the app sets isMinifyEnabled = true
, the app may crash on runtime when startSDK()
is called.
The OneTrust SDK uses Retrofit
for network calls, which requires some proguard rule updates if the app is using R8 shrinking and obfuscation rules. More info in the links below:
https://github.com/square/retrofit?tab=readme-ov-file#r8--proguard
https://github.com/square/retrofit/blob/trunk/retrofit/src/main/resources/META-INF/proguard/retrofit2.pro
To resolve the crash, add the following to the proguard-rules.pro
Option 1:
R8 full mode strips generic signatures from return types if not kept.
-if interface * { @retrofit2.http.* public *** *(...); }
-keep,allowoptimization,allowshrinking,allowobfuscation class <3>
Option 2:
Add the rule below similar to https://developer.onetrust.com/onetrust/docs/android#frequently-asked-questions-faq
-keep class retrofit2.** { *; }
Adding SDK using Maven
OneTrust SDK is published and distributed through Maven repository. Follow the below given steps to add SDK to your application as a build dependency.
- Open Gradle Scripts for your Project:
build.gradle (Project: <your_project>)
- Add the following line to the buildscript:
repositories {
mavenCentral()
}
- Add the following to the
dependencies
section of yourbuild.gradle (module: app)
file
// Implement the SDK version that corresponds to the published version
implementation 'com.onetrust.cmp:native-sdk:X.X.0.0'
// Example:
implementation 'com.onetrust.cmp:native-sdk:202209.1.0.0'
- Save and Close gradle files.
- Build your project. Once it is built successfully, you can import SDK.
Dependency Conflicts
When using Maven, the OneTrust SDK includes several dependencies that you may already include in your project. On compile, if you experience a dependency conflict, try excluding the OneTrust SDK's runtime dependency and re-build:
// Make sure the com.onetrust.cmp:native-sdk:[version] matches what you're implementing.
implementation ('com.onetrust.cmp:native-sdk:202209.1.0.0') {
exclude group: 'com.google.android.gms'
}
Import .aar
Framework
.aar
Framework- Download zip from OneTrust console which contains .aar file.
- Place the OTPublishersHeadlessSDK-release.arr inside libs folder
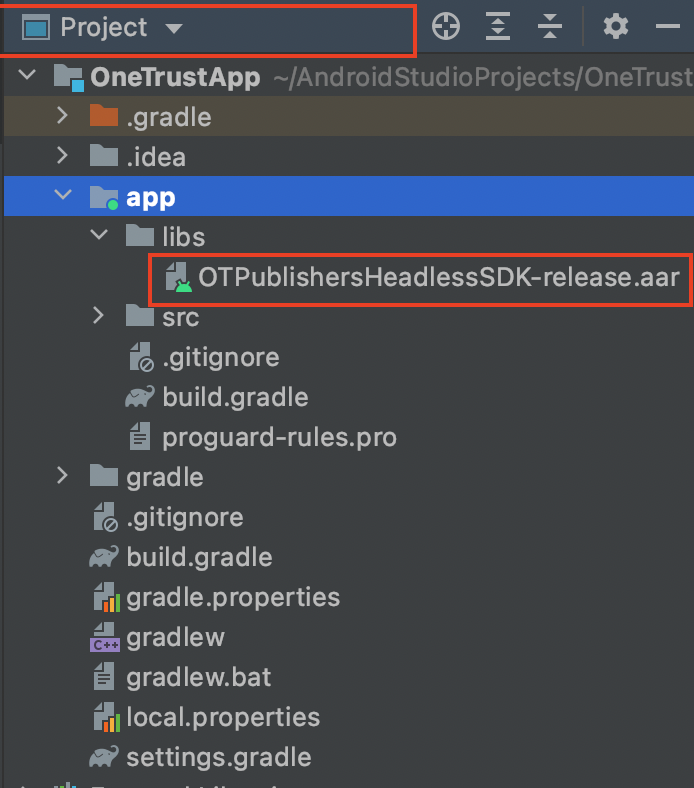
- Navigate to File > Project Structure > Dependencies
- In the Declared Dependencies tab, click + and select Jar/AAR Dependency in the dropdown
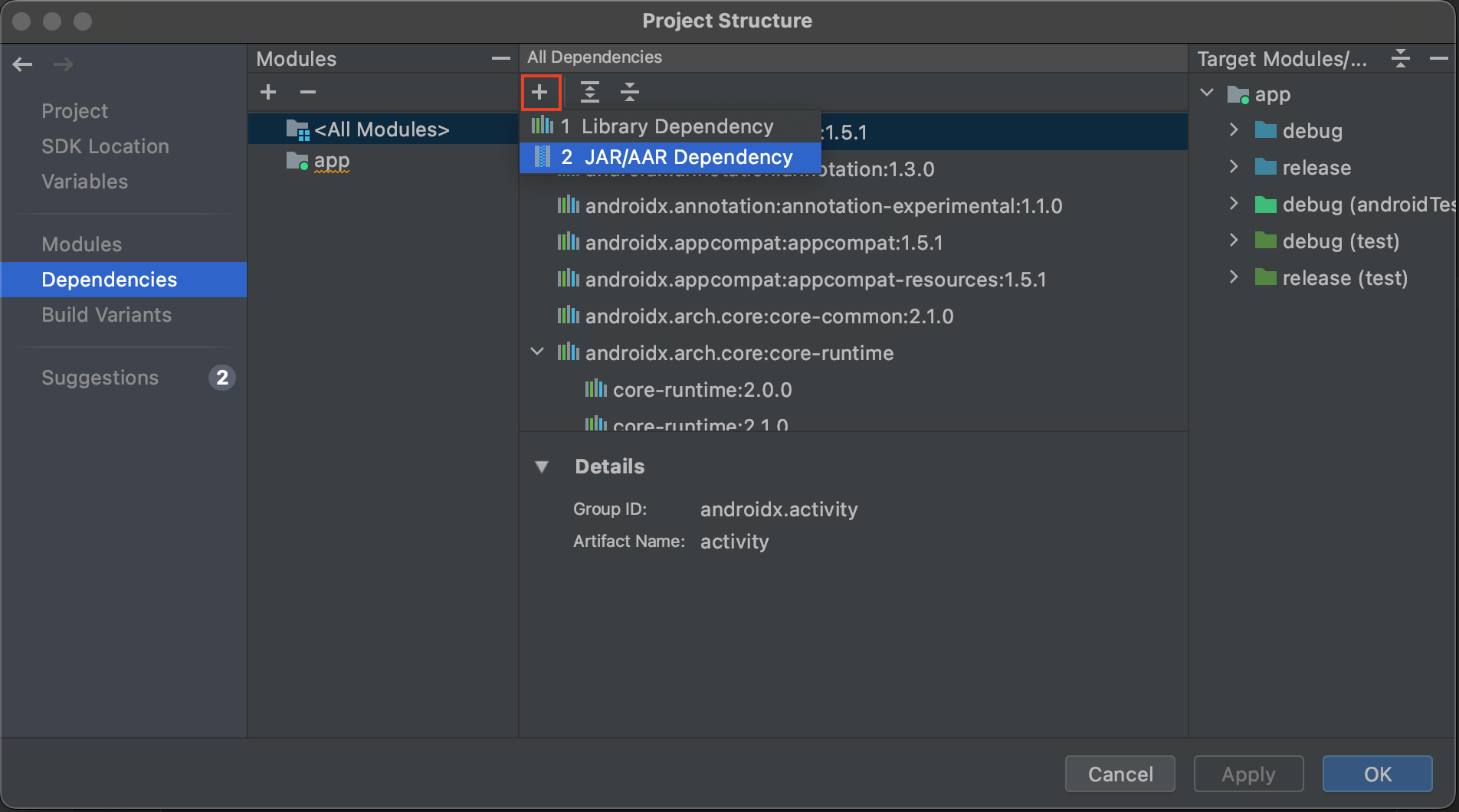
- In the Add Jar/AAR Dependency dialog, first enter the path to the OneTrust .aar file, then select the configuration implementation
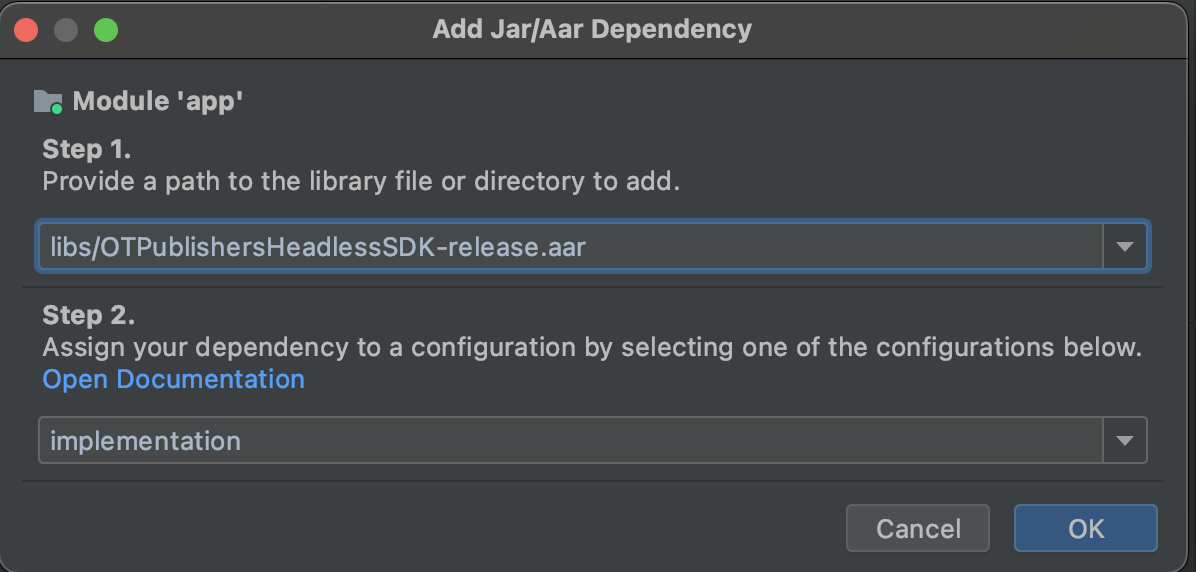
- Check your app's build.gradle file to confirm the declaration.
implementation files('libs/OTPublishersHeadlessSDK-release.arr')
- Include the dependencies (listed above) if your app has not implemented these already. Not having the dependencies will cause exceptions at runtime. We recommend you implement the latest versions.
Optional Dependencies
Google Ad Id
The Android SDK has the capability to measure the opt-in/out rates of Google's Advertising Id. According to Google, starting in late 2021 when a user opts out of interest-based advertising or ads personalization the advertising identifier will not be available and will be a string of 0's.
If you'd like to measure the opt in/out rate, it's mandatory to include the following dependency:
implementation 'com.google.android.gms:play-services-ads:7.8.0'
QR Code for Android TV
In order to show a privacy policy as a QR code on Android TV, App will have to add the following dependency manually in app's gradle file if QR code has to be supported to avoid any runtime exceptions:
implementation 'com.google.zxing:core:3.3.0'
D8 Exceptions
On compile, if you experience a any D8 exceptions with the above mentioned gradle versions, add the following code to app’s gradle file:
compileOptions {
sourceCompatibility = '1.8'
targetCompatibility = '1.8'
}
Import Header
Import header to begin using OneTrust SDK methods.
import com.onetrust.otpublishers.headless.Public.OTCallback;
import com.onetrust.otpublishers.headless.Public.OTPublishersHeadlessSDK;
Updated 3 months ago