Flutter
The OneTrust Flutter library is hosted on pub.dev.
Versioning and Installation
Versioning
The SDK version used in the app must match the version of the app data published from your OneTrust tenant. For example, if you've published version 202408.1.0 of the app data in your OneTrust environment, you must use npm package version 202408.1.0 as well. It is recommended to specify a version to avoid automatic updates, as a OneTrust publish is typically required when you update your SDK version.
Installation
To install the OneTrust plugin, run flutter pub add onetrust_publishers_native_cmp
.
Specify a version in your pubspec.yaml
file, for example:
dependencies:
onetrust_publishers_native_cmp: '202408.1.0'
This plugin pulls the OneTrust SDK from mavenCentral and Cocoapods.
After adding the plugin to your Flutter project, build the project to check for any immediate errors.
iOS Requirements
The SDK supports a minimum iOS version of 11.0. You may have to specify a platform target in your podfile
.
platform :ios, '11.0'
Android Requirements
In Android, the underlying Native SDK requires a FragmentActivity
in order to render a UI. In your Android MainActivity.java
file, ensure that the MainActivity
class is extending FlutterFragmentActivity
.
Additionally, Android must use targetSdkVersion 31
and compileSDKVersion 31
or higher.
import io.flutter.embedding.android.FlutterFragmentActivity
public class MainActivity extends FlutterFragmentActivity {
}
import io.flutter.embedding.android.FlutterFragmentActivity
class MainActivity: FlutterFragmentActivity() {
}
Android Dependency Clashes
In rare cases, one of the dependencies required by OneTrust's underlying Native Android SDK may clash with dependencies in your application. Most of the time, Gradle will resolve these automatically. If that is not the case however, OneTrust provides the option to suppress all transitive dependencies from the OneTrust .aar
file.
To suppress all transitive dependencies, add onetrust.includeTransitiveDependencies=false
to your Android project's local.properties
file. If your application suppresses OneTrust's dependencies, the dependencies must be added to your project manually. A list of required dependencies is available here.
Implementation and Development
The following sections are laid in a typical order of development and UX.
Initializing the SDK
The OneTrust SDK retrieves an object that contains all the data needed to present a UI to a user to collect consent for the application. The data returned is determined by the configurations made in the OneTrust admin console.
Before proceeding, make sure that your SDK Data has been published inside of the OneTrust Admin Portal. If the data has not been published, the SDK will not have any data to retrieve. View instructions on how to publish here.
startSDK
startSDK
This is the initialization method for the SDK. It makes between 1-2 network calls:
- 1 network call when using a non-IAB TCF template
- 2 networks calls when using a IAB TCF template
The call is an async function that returns a boolean status value:
true
Initialization and download of data was successfulfalse
Initialization and download of data was unsuccessful
Future <void> initOneTrust() async {
bool status;
Map<String, String> params = { //Params are optional
"countryCode":"US",
"regionCode":"CA"
}
try {
status = await OTPublishersNativeSDK.startSDK(
'storageLocation',
'domainIdentifier',
'en',
params);
} on PlatformException {
print("Error communicating with platform-side code");
}
if (!mounted) return;
setState(() {
_cmpDownloadStatus = status ? 'Success!':'Error';
});
}
Argument | Type | Description |
---|---|---|
storageLocation | String | The CDN location for the JSON that the SDK fetches. (Usually, but not always, cdn.cookielaw.org . |
domainIdentifier | String | The App ID to load |
languageCode | String | 2-digit or 4-digit (in the case of advanced languages) ISO language code used to return content in a specific language. Note: Any language code format which is not listed in OneTrust environment will be considered as invalid input. Note: If the languageCode passed by your application is valid, but does not match with a language configuration for your template, then the SDK will return content in the default language configured in OneTrust environment (usually, but not always, English). |
params | Map<String, String> | Parameter map to override initialization settings. Parameters are optional, but you must have a non-null object defined, even if it is blank. See params section below for more information. |
Params
All initialization parameters are expected to be of type String
, and all are optional.
Name | Type | Description |
---|---|---|
countryCode | String | 2-digit ISO country code that will override OneTrust's geolocation service for locating the user. Typically used for QA to test other regions, or if your application knows the user's location applicable for consent purposes. |
regionCode | String | 2-digit ISO region code that will override OneTrust's geolocation service. Typically used for US states (e.g. CA, GA, NC, etc.) |
androidUXParams | String | A stringified representation of the OTUXParams JSON object to override styling in-app. See "Android - Custom Styling with UXParams JSON" below. |
Get User Location
This method returns a map containing the country and state code where data was last downloaded and can be used to determine user location.
Map<String, dynamic> lastDataDownloadedLocation = await OTPublishersNativeSDK.getLastDataDownloadedLocation();
Display User Interfaces
The OneTrust SDK manages several different user interfaces to display to a user. Here's a list below for your reference:
Name | Description | Method to Show |
---|---|---|
Banner | Notice to the user of their privacy rights. Has configurable text and buttons for Accept All, Reject All, Manage Preferences, and Close Banner. Note: Each of these buttons can be toggled On/Off in the Admin Console. | showBannerUI() |
Preference Center | An interface for the user to view their current profile settings and update their choices based on the configuration provided for them. Has configurable text and buttons for Accept All, Reject All, Save Settings, and Close Preference Center. Note: You can choose to hide each button except Save Settings - this is required for a User to update their choices. | showPreferenceCenterUI() |
Purpose Details | The Purpose Details (or Category Details) view shows granular detail about the category and also shows the SDK LIst link, Vendor List link, and child categories based on configuration. | none, sub-view of Preference Center |
SDK List | An interface to show a granular list of SDKs to the user. This list may be filtered by Category to provide more transparency to the User. Note: This can be hidden in Template Settings in the Admin Console. | none, sub-view of Preference Center |
IAB Vendor List | An interface, shown only for IAB2 type templates. Displays a list of 3rd party ad tech vendors under management by the application. This provides a way for users to opt in/out of consent for a particular vendor. | none, sub-view of Preference Center |
The user interfaces without methods indicate they can only be navigated to based on user navigation and admin portal settings.
showBannerUI
showBannerUI
This method will always show the Banner UI, regardless of the shouldShowBanner
value.
OTPublishersNativeSDK.showBannerUI();
showPreferenceCenterUI
showPreferenceCenterUI
This method will always show the Preference Center UI.
OTPublishersNativeSDK.showPreferenceCenterUI();
shouldShowBanner
shouldShowBanner
This method determines if a Banner UI should be displayed based on SDK determined logic. Returns a boolean.
bool _shouldShowBanner;
try {
_shouldShowBanner = await OTPublishersNativeSDK.shouldShowBanner();
} on PlatformException {
print("Error communicating with platform-side code");
}
if(_shouldShowBanner){
OTPublishersNativeSDK.showBannerUI();
}
How is shouldShowBanner()
computed?
-
Is the geolocation rule configured to show a banner? This is controlled by the
showAlertNotice
property in the JSON beingtrue
.- If
showAlertNotice = false
, the method returnsfalse
and a banner should not be shown for this region. - If
showAlertNotice = true
, move to the next check.
- If
-
Was the most recent SDK Publish configured to re-consent users? This is controlled by the presence of 2 values. 1) A
LastReconsentDate
property in JSON with non-null value. 2) AOneTrustLastReconsentDate
value saved on disk- If the
LastReconsentDate
JSON is newer than theOneTrustLastReconsentDate
on disk, the method returnstrue
and a banner should be shown. - If no, move to the next check
- If the
-
Is the geolocation rule configured for automatic re-consent AND did the user's automatic re-consent timer expire? This is controlled by measuring the difference between
OneTrustLastConsentDate
saved on disk and theCurrent Date
and seeing if it is greater than or equal toOneTrustReconsentFrequencyDays
in the JSON saved on disk. The SDK considers the date itself and not the time consent was given. For example, if the re-consent period is set to 1 day in my geolocation rule and the user provides consent at 11:59pm GMT, the banner will reappear at 12:00am GMT (1 minute later, but the next day).- If yes, the method returns
true
and a banner should be shown. - If no, move to the next check
- If yes, the method returns
-
Is Cross Device Consent enabled and were 100% of Purposes synced? This is controlled by the
profile.sync.allPurposesUpdatedAfterSync
property in JSON beingtrue
.- If yes, the method returns
false
and a banner should not be shown. This is because each of the Categories/Purposes managed by the SDK were 100% synced from the user's profile on the OneTrust server. - If no, move to the next check.
- If yes, the method returns
-
Is Cross Device Consent enabled and is the user being exposed to new Categories/Purposes since the last time their consent was saved? This would only occur if Cross Device Consent is enabled and there was a new publish to the SDK which exposes new Categories/Purposes that have at least 1 SDK assigned to it.
- If yes, the method returns
true
and a banner should be shown. - If no, move to the next check.
- If yes, the method returns
-
Has the user previously given consent and is that consent stored at disk?
- If yes, the method returns
false
and a banner should not be shown. - If no, the method returns
true
and a banner should be shown because this is the first time they are being asked to give consent on the app.
- If yes, the method returns
-
Has a new category been added post giving consent?
- If yes, the method returns
true
and a banner should be shown again because user needs to give consent to the new category. - If no, the method returns
false
and a banner should not be shown.
- If yes, the method returns
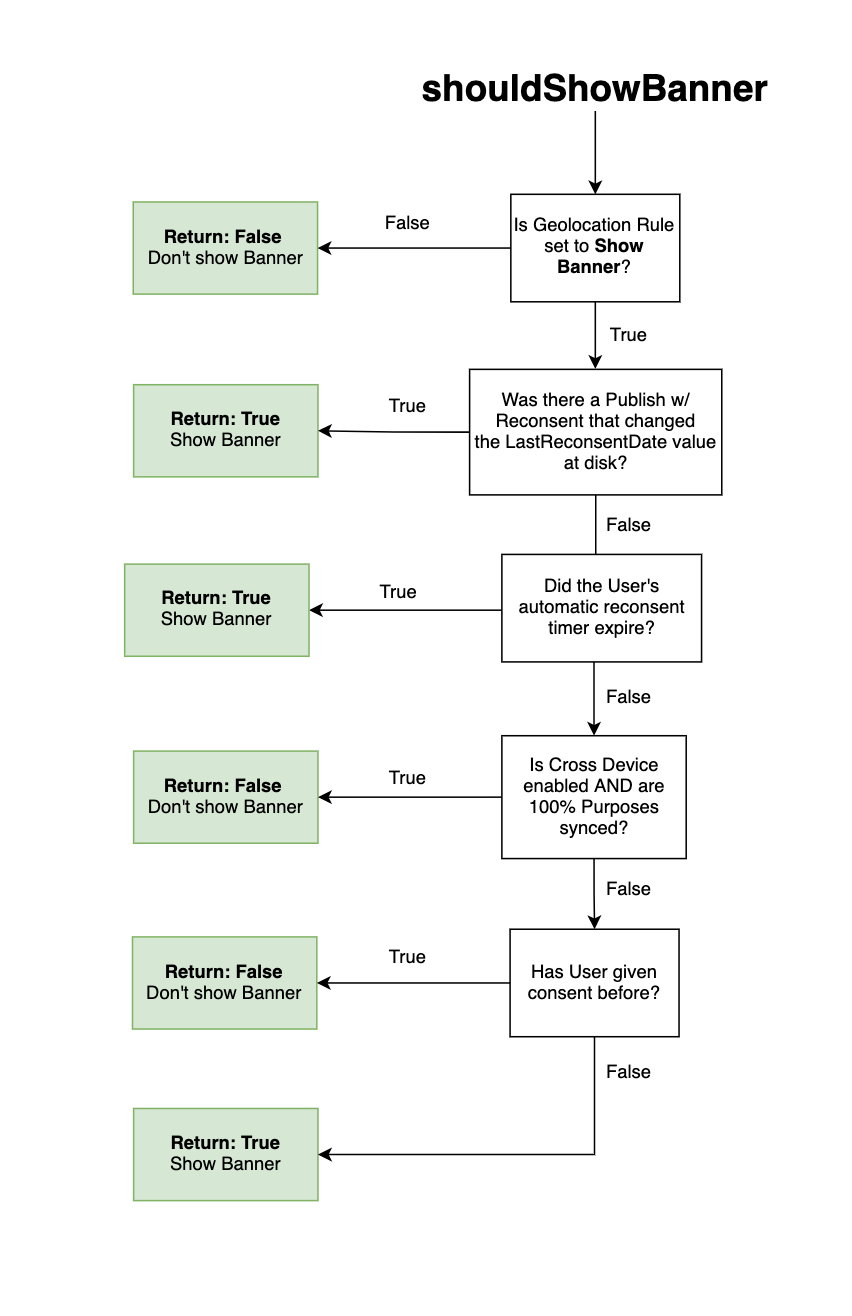
When Consent Changes
When a user updates their consent choices or interacts with a OT SDK UI, the Application needs to become aware of that so it can control the next set of actions like:
- Allowing or Restricting processing of 3rd party SDKs based on their categorization
- Passing latest User consent values to 3rd Party SDKs
- Passing the IAB’s encoded TC String to 3rd party SDKs
- Passing the IAB’s USP String to 3rd Party SDKs
- Routing the User to another location in the App
- Displaying messages to the User of their changes
- Logging updates to internally owned APIs
- etc.
Consent Values
OneTrust universally uses the following values for consent status:
Consent value | Description |
---|---|
1 | Consent given |
0 | Consent not given |
-1 | Consent not yet gathered, or SDK not initialized |
Listening for Consent Changes
The OneTrust SDK broadcasts consent events when you call startSDK()
and when consent values change. You can use these events and consent values to trigger some action. Your app can listen to either the categories itself or each individual SDK you want to manage. OneTrust recommends setting up observers on the category level to minimize the amount of observers you have to initialize.
Use Case: Applications needs observers for the SDKs or Categories that require some action to be taken when a user gives/withdraws their consent.
Example: When a user opts out of the "Sale of Personal Data" category, set a privacy or opt out flag in Targeting SDKs in scope so no personalized ads or ads of any kind are shown to the user.
The Flutter library implements an EventChannel
that opens a BroadcastStream
with the platform-side code to listen for changes to the consent state.
var consentListener = OTPublishersNativeSDK.listenForConsentChanges(["C0002","C0003"]).listen((event) {
setCategoryState(event['categoryId'], event['consentStatus']);
print("New status for " + event['categoryId'] + " is " + event['consentStatus'].toString());
});
consentListener.cancel() //destroy the listener
Note that:
- The
BroadcastStream
will stay open until it is closed by calling.cancel()
on it - Only one
BroadcastStream
perEventChannel
can be open at a single time. Therefore, you must call.cancel()
on this stream before calling.listenForConsentChanges().listen
again.
This listener accepts an array of strings containing Category IDs to listen for. The listener returns an object:
{
'categoryId':String
'consentStatus':int
}
OTConsentUpdated
The SDK will also broadcast a generic event every time a OneTrust UI (banner or preference center) is dismissed.
Use case: Applications need to know when they can query for latest consent for vendors, categories, SDKs, etc. This is useful in cases where you do not or cannot set up multiple observers for each category or SDK.
Event name: OTConsentUpdated
Querying for Consent
Query the current consent status for any of the categories or SDKs included in your application. This can be used to determine what privacy action is needed at app launch or anytime the consent status is needed without being notified by an event broadcast. Simply pass in the Category ID (eg. C0001) or SDK ID and the method will return the current consent status (integer value).
Querying Category Consents
Future <void> getConsentStatus() async {
int status;
try{
status = await OTPublishersNativeSDK.getConsentStatusForCategory("Category ID");
} on PlatformException {
print("Error communicating with platform-side code.");
}
print("Queried Status for Category is = " + status.toString());
}
Querying SDK Consents
Future <void> getConsentStatus() async {
int status;
try{
status = await OTPublishersNativeSDK.getConsentStatusForSDK("SDK ID");
} on PlatformException {
print("Error communicating with platform-side code.");
}
print("Queried Status for SDK is = " + status.toString());
}
UI Interaction Events
Note
UI Interaction Events are only relevant if the app is using the out of the box OneTrust UI. If you have built your own UI, disregard this section.
When a User interacts with the OT SDK UI, the SDK sends an interaction event that the application can listen for and action on.
Use Case: The app needs to trigger certain actions based on interaction with the UI.
Use Case 2: In the event that broadcasts cannot be used to action on consent, the app can rely solely on interaction events and querying for consent to fire/suppress SDKs.
Example 1: User selects the "Confirm My Choices" button on the OT Preference Center UI, which saves their consent choices. An app might want to listen for the onPreferenceCenterConfirmChoices() to show a success toaster to the user and navigate them to the App's home page.
Example 2: In lieu of broadcast events, the app can listen for allSDKViewsDismissed to know when the OneTrust UI has been dismissed and query for user consent. It can then use this consent to fire/suppress SDKs.
The Flutter library implements an EventChannel
that opens a BroadcastStream
with the platform-side code to listen for changes in the UI.
var interactionListener = OTPublishersNativeSDK.listenForUIInteractions().listen((event) {
print(event);
});
Note that:
- The
BroadcastStream
will stay open until it is closed by calling.cancel()
- Only one
BroadcastStream
perEventChannel
can be open at a single time. Therefore, you must call.cancel()
on this stream before calling.listenForUIInteractions().listen
again.
Expected return:
{
'uiEvent':String,
'payload':Object
}
Types of Events:
Event Name | Description | Payload |
---|---|---|
onShowBanner | Triggered when banner is shown | null |
onHideBanner | Triggered when banner is closed | null |
onBannerClickedAcceptAll | Triggered when user allows all consent from banner | null |
onBannerClickedRejectAll | Triggered when user rejects all consent from banner | null |
onShowPreferenceCenter | Triggered when Preference Center is displayed | null |
onHidePreferenceCenter | Triggered when Preference Center is closed | null |
onPreferenceCenterAcceptAll | Triggered when user allows all consent from Preference Center | null |
onPreferenceCenterRejectAll | Triggered when user rejects all consent from Preference Center | null |
onPreferenceCenterConfirmChoices | Triggered when user clicked on save choices after updating consent values from Preference Center | null |
onShowVendorList | Triggered when vendor list UI is displayed from an IAB banner/ IAB Preference center | null |
onHideVendorList | Triggered when vendor list UI is closed or when back button is clicked | null |
onVendorConfirmChoices | Triggered when user updates vendor consent / legitimate interests purpose values and save the choices from vendor list | null |
onVendorListVendorConsentChanged | Triggered when user updates consent values for a particular vendor id on vendor list UI | {vendorId:String, consentStatus:Int} |
onVendorListVendorLegitimateInterestChanged | Triggered when user updates Legitimate interests values for a particular vendor id on vendor list UI | {vendorId:String, legitInterest:Int} |
onPreferenceCenterPurposeConsentChanged | Triggered when user updates consent values for a particular category on Preference Center UI | {purposeId:String, consentStatus:Int} |
onPreferenceCenterPurposeLegitimateInterestChanged | Triggered when user updates Legitimate interest values for a particular category on Preference Center UI | {purposeId:String, legitInterest:Int} |
allSDKViewsDismissed | Triggered when all the OT SDK Views are dismissed from the view hierarchy. | {interactionType:String} |
Clear SDK Consents and Data
This method clears all data from the SDK:
OTPublishersNativeSDK.clearOTSDKData();
Special Configurations
Passing Consent to WebViews
If your application uses WebViews to present content and the pages rendered are running the OneTrust Cookies CMP, you can inject a JavaScript variable, provided by SDK, to pass consent from the native application to your WebView.
The JavaScript must be evaluated before the Cookies CMP loads in the webview. Therefore, it is recommended to evaluate the JavaScript early on in the WebView load cycle.
getOTConsentJSForWebview
getOTConsentJSForWebview
String jsToPass = await OTPublishersNativeSDK.getOTConsentJSForWebview();
Example output:
var OTExternalConsent = {
"USPrivacy": "1---",
"addtlString": "",
"consentedDate": "Tue, 6 Apr 2021 16:13:41 +0100",
"groups": "C0002:1,C0003:1,C0005:0,C0004:0,C0001:1",
"tcString":"CPEPMp6PEPMp6AcABBFRBUCgAAAAAAAAAChQHrAA...",
"gppString":"DBABzw~1YNY~BVQqAA...."
}
Important
It's recommended that the application call getOTConsentJSForWebview
when you need to render a WebView. This ensures the payload is up to date based on the user's latest consent.
However, if you have a use case to call this method after consent has been changed by the user on the app, you can utilize the OTConsentUpdated
broadcast from the SDK. This event is broadcast any time a OneTrust UI has been dismissed.
Do not rely on the broadcasts for category events. Due to some order of operations under the hood, the JS variable above may not be updated by the time you call getOTConsentJSForWebview
.
You can view more information (including FAQs) in the native documentation here: iOS | Android
Programmatically Update Consent
When using the out of the box UI, the SDK will handle updates to the consent status. However, the app can programmatically update a consent value for a category (e.g. C0002, C0003, etc) if needed. Modifying the user's consent is a two step approach. updatePurposeConsent
is used to set the state of the consent (toggles) on the UI and saveConsent
is used to commit and save the consent to local storage. saveConsent
MUST be called or consent will NOT be committed and changed.
To update the consent status of a category:
OTPublishersNativeSDK.updatePurposeConsent("Category ID", true);
Parameter | Type | Description |
---|---|---|
Category ID | String | ID of the category to be updated |
Consent value | Boolean | Consent status given by the user for a category/group |
To save and log the changes:
OTPublishersNativeSDK.saveConsent(OTInteractionType.preferenceCenterConfirm);
Interaction Types | Description |
---|---|
bannerAllowAll | User has accepted all on the banner. |
bannerRejectAll | User has rejected all on the banner. |
bannerContinueWithoutAccepting | User has selected the "Continue Without Accepting" CTA on the banner. |
bannerClose | User has selected the 'x' on the banner. |
preferenceCenterAllowAll | User has selected Allow All in the preference center. |
preferenceCenterRejectAll | User has selected Reject All in the preference center. |
preferenceCenterConfirm | User has selected Confirm in the preference center. |
Apple App Tracking Transparency and Age Gate
If enabled in your template, OneTrust can render a pre-prompt UI and then show the App Tracking Transparency prompt immediately after. You can view more information about this here. Returns an integer.
showConsentUI
showConsentUI
/int authStatus = await OTPublishersNativeSDK.showConsentUI(OTDevicePermission.idfa);
//Use the following to get the status as an enum -- there are several outcomes for IDFA specifically
OTATTrackingAuthorizationStatus IDFAStatus = OTATTrackingAuthorizationStatus.values[authStatus];
This method will not resolve until the user has made a selection within the native iOS App Tracking Transparency prompt. It takes an argument of type OTDevicePermission
which is provided as an enum
for ease of use. It returns an OTATTrackingAuthorizationStatus
.
Query ATT Status
The current status of App Tracking Transparency can be obtained by calling:
OTATTrackingAuthorizationStatus authStatus = await OTPublishersNativeSDK.getATTrackingAuthorizationStatus();
This method returns an OTATTTrackingAuthorizationStatus
which is namespaced to avoid issues with other ATT plugins in use. For unsupported OS versions (all Android versions and iOS versions < 14,) this function will return platformNotSupported
. Possible values of the enum are:
notDetermined
restricted
denied
authorized
platformNotSupported
Age Gate
Additionally, OneTrust can also render an age gate prompt.
//Age Gate - iOS and Android
int authStatus = await OTPublishersNativeSDK.showConsentUI(OTDevicePermission.AgeGate);
//returns a 0 (user responded no) or a 1 (user responded yes)
To query for the consent status of the age gate prompt:
int authStatus = await OTPublishersNativeSDK.getAgeGatePromptValue();
Returns a 0
for a No response and a 1
for a Yes response.
Customize User Interfaces
This section is optional as most UI configurations are primarily made through the OneTrust tenant. If your app has a use case where it needs make additional configurations (e.g. font family) otherwise not available in the tenant OR if it needs to control the UI configurations locally, you can use these custom configurations.
Custom Fonts
Custom Fonts are only supported on iOS through Flutter. Android does not support Custom Fonts through uxParams.
Android - Custom Styling with uxParams JSON
OneTrust allows you to add custom styling to the UI by passing in a formatted JSON. Build out your JSON by following the guide in the OneTrust Developer Portal.
Pass the JSON as a string into the startSDK
function's params
argument.
String AndroidUXParams = await DefaultAssetBundle.of(context)
.loadString("assets/AndroidUXParams.json");
Map<String, String> params = {
"androidUXParams": AndroidUXParams
};
try {
status = await OTPublishersNativeSDK.startSDK(
"cdn.cookielaw.org", appId, "en", params);
} on PlatformException {
print("Error communicating with platform code");
}
iOS - Custom Styling with uxParams Plist
Custom styling can be added to your iOS app by using a .plist file in the iOS platform code. In addition to adding the .plist file (which can be obtained from the OneTrust Demo Application) to your bundle, there are a few changes that need to be made in the platform code, outlined below. Review the guide in the OneTrust Developer Portal.
In AppDelegate.swift
, import OTPublishersHeadlessSDK. Add an extension below the class to handle the protocol methods:
import OTPublishersHeadlessSDK
...
extension AppDelegate: UIConfigurator{
func shouldUseCustomUIConfig() -> Bool {
return true
}
func customUIConfigFilePath() -> String? {
return Bundle.main.path(forResource: "OTSDK-UIConfig-iOS", ofType: "plist")
}
}
In the didFinishLaunchingWithOptions
protocol method, assign the AppDelegate as the UIConfigurator:
@objc class AppDelegate: FlutterAppDelegate {
override func application(
_ application: UIApplication,
didFinishLaunchingWithOptions launchOptions: [UIApplication.LaunchOptionsKey: Any]?
) -> Bool {
OTPublishersHeadlessSDK.shared.uiConfigurator = self //add this line only
GeneratedPluginRegistrant.register(with: self)
return super.application(application, didFinishLaunchingWithOptions: launchOptions)
}
}
Google Consent Mode
Google Consent Mode allows web and app developers to adjust tag and app SDK behavior based on user consent choices. Google will be able to dynamically adapt the behavior of Google tags, Google Analytics, Google Ads, Floodlight and Conversion Linker. For more information about how this works, view these docs for the Native SDK: iOS | Android
getOTGoogleConsentModeData
allows you to retrieve the consent status for Google's consent types:
Map<String, dynamic>? googleConsentModeData = await OTPublishersNativeSDK.getOTGoogleConsentModeData();
print("getOTGoogleConsentModeData = $googleConsentModeData");
String? analyticsStorageStatus = googleConsentModeData?["analytics_storage"];
print("analyticsStorageStatus = $analyticsStorageStatus");
Set and Get Data Subject Identifier
By default, OneTrust sets a random GUID for all users. You can use the methods below to retrieve the current identifier as well as set your own.
Get Current Data Subject Identifier
String id = await OTPublishersNativeSDK.getCurrentActiveProfile();
Set Data Subject Identifier
await OTPublishersNativeSDK.renameProfile(null, "new identifier")
await OTPublishersNativeSDK.renameProfile("old identifier", "new identifier")
- First argument: Current Identifier
- Second argument: New Identifier
If the current identifier is the OneTrust random GUID default, pass in 'null' to the first argument.
This method will return true
to indicate the rename was successful. Otherwise, a FlutterError will be thrown. For example, if a profile of that name already exists, an error will be thrown.
Bring Your Own UI
The SDK provides public methods that can be leveraged to support a BYOUI experience.
Get Domain Data
This method returns a map containing all the information about the application including rule sets, template and geolocation rule configurations.
Map<String, dynamic> domainInfo = await OTPublishersNativeSDK.getDomainInfo();
Get Common Data
This method returns a map which contains template based information. This includes information like branding which includes keys for determining colors and styles for the UI specific to a template configured for the user's geolocation along with consent logging information.
Map<String, dynamic> commonData = await OTPublishersNativeSDK.getCommonData();
Get Groups Data
This method returns a dictionary containing the information about all the categories and SDK IDs specific to a template configured for the user's geolocation. This is generally used to populate the preference center's toggle section.
Map<String, dynamic> groups = await OTPublishersNativeSDK.getDomainGroupData();
Get Banner Data
This method returns a dictionary which contains all the keys required to render a banner UI.
Map<String, dynamic> bannerData = await OTPublishersNativeSDK.getBannerData();
Get Preference Center Data
This method returns a dictionary which contains all the keys required to render a preference center UI.
Map<String, dynamic> preferenceCenterData = await OTPublishersNativeSDK.getPreferenceCenterData();
Get Vendor Details
This method returns a dictionary which contains all the details for vendor.
Map<String, dynamic>? iabVendorDetails = await OTPublishersNativeSDK.getVendorDetails("vendor id", "vendorListMode");
Programmatically Update and Save Consent
Modifying the user's consent is a two step approach. updatePurposeConsent()
is used to set the state of the consent (toggles) on the UI and saveConsent()
is used to commit and save the consent to local storage. saveConsent()
MUST be called or consent will NOT be committed and changed.
OTPublishersNativeSDK.updatePurposeConsent("C0002", true);
OTPublishersNativeSDK.updatePurposeConsent("C0004", false);
OTPublishersNativeSDK.saveConsent(OTInteractionType.preferenceCenterConfirm);
OTPublishersNativeSDK.saveConsent(OTInteractionType.bannerAllowAll);
Possible OTInteractionType
values:
Interaction Types | Description |
---|---|
bannerAllowAll | User has accepted all on the banner. |
bannerRejectAll | User has rejected all on the banner. |
bannerContinueWithoutAccepting | User has selected the "Continue Without Accepting" CTA on the banner. |
bannerClose | User has selected the 'x' on the banner. |
preferenceCenterAllowAll | User has selected Allow All in the preference center. |
preferenceCenterRejectAll | User has selected Reject All in the preference center. |
preferenceCenterConfirm | User has selected Confirm in the preference center. |
Reset Updated Consent Values for All Categories
This method resets any updated Category/Purpose consent values to their defaults prior to being saved to disk. Use this method if a user makes updates in their Preference Center but then exits without saving their choices.
OTPublishersNativeSDK.resetUpdatedConsent();
Universal Consent Purposes
Important
Universal Consent Purposes for Mobile is part of the UCPM offering and you must have a UCPM license to use this module.
Universal Consent can be used to serve a different use case compared to the regular Mobile App Consent banner and this can also be configured within the Mobile SDK. Rather than collecting user consent to help manage SDKs and other technologies on the app, Universal Consent helps collect consent for purposes like promotional emails, newsletters, SMS messaging, etc. that typically require an integration with a third party system like Salesforce and Marketo. After a Universal Consent transaction is collected, integration workflows can be triggered within the OneTrust tool.
Universal Consent requires a user identifier to work properly. In order to set a data subject and keep their consent in sync with other collection methods, utilize the guidance for cross-device consent above.
Display Universal Consent Preference Center
To load the Universal Consent preference center over the current screen:
OTPublishersNativeSDK.showUCPurposesUI()
Query for Consent Values
This plugin exposes async methods to retrieve the current state of the users' consent.
To query for purpose consent:
int? consent = await OTPublishersNativeSDK.getUCPurposeConsent('purposeId')
Argument | Type | Description |
---|---|---|
purposeId | String | The GUID of the purpose to retrieve. |
Value returned:
Status | Explanation |
---|---|
1 | Consent is given |
0 | Consent is not given |
-1 | Consent not yet gathered, or SDK not initialized |
To query for a custom preference nested under a purpose:
int? consent = await OTPublishersNativeSDK.getUCPurposeCustomPreferenceOptionConsent('customPreferenceOptionId',
'customPreferenceId','purposeId')
Argument | Type | Description |
---|---|---|
customPreferenceOptionId | String | The GUID of the custom preference option |
customPreferenceId | String | The GUID of the custom preference group |
purposeId | String | The GUID of the purpose under which the custom preference is nested. |
Value returned:
Status | Explanation |
---|---|
1 | Consent is given |
0 | Consent is not given |
-1 | Consent not yet gathered, or SDK not initialized |
Programmatically Set Consent Values
The package exposes methods to programmatically set the users' consent values. A common use case is when a sign-up form has a checkbox at the bottom to allow the user to opt into emails. In this case, the application would set the value of the associated purpose, and the user could change his or her decision later in the preference center.
After making consent updates, the application must call the saveUCConsent()
function to commit the changes.
To update the top-level purpose's consent:
await OTPublishersNativeSDK.updateUCPurposeConsent('purposeId', true)
Argument | Type | Description |
---|---|---|
purposeId | String | The GUID of the purpose to update |
consent | Boolean | Whether or not consent has been granted for the specified purpose |
To update a custom preference nested under a purpose:
OTPublishersNativeSDK.updateUCPurposeCutomPreferenceOptionConsent('customPreferenceOptionId',
'customPreferenceId','purposeId', true)
Argument | Type | Description |
---|---|---|
customPreferenceOptionId | String | The GUID of the custom preference option |
customPreferenceId | String | The GUID of the custom preference group |
purposeId | String | The GUID of the purpose under which the custom preference is nested. |
consent | Boolean | Whether or not consent has been granted for the specified item |
Save Consent
After making updates to the consent values, the application must call the following method to commit the changes:
OTPublishersNativeSDK.saveConsent(OTInteractionType.ucPreferenceCenterConfirm)
Updated 12 days ago