Initialize SDK
Overview
The OneTrust SDK retrieves an object that contains all the data needed to present a UI to a User to collect consent for the SDKs used in your application. The data returned is determined by the configurations made in the OneTrust Admin console.
Before proceeding, make sure that your SDK Data has been published inside of the OneTrust Admin Portal. If the data has not been published, the SDK will not have any data to retrieve. View instructions on how to publish here.
startSDK
startSDK
Important
Note: As of 6.15.0, the
initOTSDKData()
method is deprecated and should be replaced withstartSDK()
. You can read more about the reasons why here.
This method is the initialization method for the SDK. It makes between 1-2 network calls:
- 1 network call when using a non-IAB TCF2 implementations
- 2 networks calls when using a IAB TCF2 template
OTPublishersHeadlessSDK otPublishersHeadlessSDK = new OTPublishersHeadlessSDK(this);
// more info on the input params below
otPublishersHeadlessSDK.startSDK(domainURL, domainId, languageCode, sdkParams, false, new OTCallback() {
@Override
public void onSuccess(@NonNull OTResponse otSuccessResponse) {
// do logic to render UI
String otData = otSuccessResponse.getResponseData();
}
@Override
public void onFailure(@NonNull OTResponse otErrorResponse) {
// Use below method to get errorCode and errorMessage.
int errorCode = otErrorResponse.getResponseCode();
String errorDetails = otErrorResponse.getResponseMessage();
// Use toString() to log complete OT response
Log.i(LOG_TAG, otErrorResponse.toString());
}
});
Successful initialization
After the SDK is successfully initialized at least once, it caches the data locally on the device. If the initialization fails the next time around, users will still be able to access the CMP UI and make consent choices.
Parameters
These are the parameters for the startSDK()
method.
Parameter | Description | Required |
---|---|---|
domainUrl | The CDN location for the JSON that the SDK fetches. (Usually, but not always, cdn.cookielaw.org . | Yes |
domainId | The Application guid (retrieved from OneTrust Admin console) | Yes |
languageCode | 2-digit or 4-digit (in the case of advanced langauges) ISO language code used to return content in a specific langauge. Note: Any language code format which is not listed in OneTrust environment will be considered as invalid input. Note: If the languageCode passed by your application is valid, but does not match with a language configuration for your template, then the SDK will return content in the default language configured in OneTrust environment (usually, but not always, English). | Yes |
sdkParams | A parameter of type OTSdkParams which contains other optional SDK params. See below for more details. | No |
loadOffline | Set to true to load Offline Mode data. | No |
OTCallback | A callback that fires once the SDK's fetch process completes. | Yes |
Response Object
This is the return of the startSDK()
method. It is OTResponse
type.
Method | Description | Type |
---|---|---|
getResponseCode() | Represents an integer value for the HTTP response code. | Int |
getResponseType() | Represents a success or error response type. | String |
getResponseMessage() | Represents details about the response. | String |
getResponseData() | Represents the entire server response received while starting OT SDK. | String |
What should the domainUrl
value be?
domainUrl
value be?The domainUrl
paramter is the CDN Location
value which you retrieve from the OneTrust Admin under SDKs > {Select App from List} > Instructions (pictured below). This value is OneTrust environment dependent and the potential values can also be found using the Location header table on the Mobile App Data API reference.
What should the domainId
value be?
domainId
value be?The domainId
parameter is the Mobile App ID
value which you retrieve from the OneTrust Admin under SDKs > {Select App from List} > Instructions (pictured below).
OTSdkParams
OTSdkParams
OTSdkParams sdkParams = OTSdkParams.SdkParamsBuilder.newInstance()
.setOTCountryCode("US")
.setOTRegionCode("CA")
.shouldCreateProfile("false")
.setProfileSyncParams(otProfileSyncParams) // otProfileSyncParams explained below
.build();
Parameter | Description | Release Version |
---|---|---|
setOTCountryCode | Two-letter ISO 3166-2 country code. This can be used to bypass the automatic geo-ip lookup performed by the SDK. | 6.4.0 |
setOTRegionCode | Two-letter state code, used alongside countryCode, typically used for CCPA use cases when providing an experience for California-based US citizens. | 6.4.0 |
shouldCreateProfile | When set to true , this will tell the SDK to create a Data Subject Profile in the Universal Consent module. Note: This should only be used for applications utilizing the Cross Device Consent functionality. Please contact your OneTrust Consultant for more information on this feature. | 6.5.0 |
setProfileSyncParams | When passed in, the SDK will attempt to sync and update a User's Profile based on the latest changes. This is known as Cross Device Consent and is an optional feature. Note: This should only be used for applications utilizing the Cross Device Consent functionality. Please contact your OneTrust Consultant for more information on this feature. | 6.7.0 |
Passing Custom Geolocation
The OneTrust SDK, by default, determines a country and region code for a User based on a geo-ip lookup when calling startSDK
. If you choose to perform your own geolocation lookup and override the OneTrust computed one, this is supported by using the countryCode
and regionCode
parameters mentioned above.
Retrieving User Location
The SDK returns the location where data was last downloaded and can be used to determine user location. Returns a string.
OTPublishersHeadlessSDK otPublishersHeadlessSDK = new OTPublishersHeadlessSDK(this);
otPublishersHeadlessSDK.getLastDataDownloadedLocation().country;
otPublishersHeadlessSDK.getLastDataDownloadedLocation().state;
The SDK returns the location where the user last interacted with the SDK UI and provided consent. Returns a string.
OTPublishersHeadlessSDK otPublishersHeadlessSDK = new OTPublishersHeadlessSDK(this);
otPublishersHeadlessSDK.getLastUserConsentedLocation().country;
otPublishersHeadlessSDK.getLastUserConsentedLocation().state;
Configuring Banner Height
By default, the OneTrust SDK renders the Banner in full screen. You can update this to instead be Two-thirds
, Half
, or One-third
depending on preference.
Recommended Way: OneTrust recommends configuring the Banner Height from the OneTrust Admin via Templates > Mobile App > Banner > General. This way it can be updated via Publish instead of being hard-coded to the application and require a version update to App Stores.
Legacy Way: Set the OTBannerHeightRatio
parameter to either OTBannerHeightRatio.TWO_THIRD
, OTBannerHeightRatio.ONE_HALF
, or OTBannerHeightRatio.ONE_THIRD
.
setOTOfflineData()
setOTOfflineData()
As of 202209.1.0, we have introduced an offline data mode which can be used to load the OneTrust SDK offline.
Note: Make sure to call this API before calling startSDK with loadOffline parameter as true.
If the loadOffline parameter is passed as false but there is no internet connectivity while making the network call to OneTrust, the OneTrust SDK will try using the offline data that is setup using otPublishersHeadlessSDK.setOfflineData()
. If the data is not available, then it will fail to initialize the OneTrust SDK.
In order to retrieve the main SDK JSON needed for offline mode, you will have to fetch the data using our mobile data endpoint: https://developer.onetrust.com/onetrust/reference/applicationdata.
Note
The endpoints below are only needed if your app is using IAB TCF Framework. If you're not using Google Additional Vendors, disregard the Google endpoint.
- IAB TCF Vendor List Endpoint: https://cdn.cookielaw.org/vendorlist/iab2Data.json
- Google Vendor List Endpoint: https://cdn.cookielaw.org/vendorlist/googleData.json
After retrieving the JSON specific to your App ID, you'll supply this to the SDK.
Reading Asset File - Code Snippet
@Nullable
String readAssetFile(@NonNull Context context, @NonNull String fileName) {
String fileContent = null;
InputStream inputStream = null;
try {
inputStream = context.getAssets().open(fileName);
int size = inputStream.available();
byte[] buffer = new byte[size];
int isRead = inputStream.read(buffer);
Log.v(LOG_TAG, "bytes read for Asset file : " + isRead);
inputStream.close();
fileContent = new String(buffer);
} catch (IOException e) {
Log.e(LOG_TAG, "File read error = " + e.getMessage());
} finally {
if (inputStream != null) {
try {
inputStream.close();
} catch (IOException e) {
Log.e(LOG_TAG, "Error on closing FileInputStream message = " + e.getMessage());
}
}
}
return fileContent;
}
Set Banner and Preference Center Logo
public OTConfiguration getOTConfiguration(@NonNull Context context) {
OTConfiguration.OTConfigurationBuilder otConfigurationBuilder = OTConfiguration.OTConfigurationBuilder.newInstance();
otConfigurationBuilder = otConfigurationBuilder.setBannerLogo(logo_location);
otConfigurationBuilder = otConfigurationBuilder.setPCLogo(logo_location);
OTConfiguration otConfig = otConfigurationBuilder.build()
}
// pass the otConfig instance as a param to the UI methods - showBannerUI()/setupUI()/showPreferenceCenterUI()
new OTPublishersHeadlessSDK(this).showBannerUI(RenderNativeUIActivity.this, otConfig);
Non-IAB TCF
Use the code below for a standard GDPR, CCPA and IAB CCPA implementation:
private void setOfflineData() throws JSONException {
JSONObject offlineData = new JSONObject();
String otData = readAssetFile(this, "ot_offline_domain_data.json");
if (!TextUtils.isEmpty(otData)) {
offlineData.put("otData", new JSONObject(otData));
}
new OTPublishersHeadlessSDK().setOTOfflineData(offlineData);
}
// Must call setOfflineData() first before startSDK()
setOfflineData()
startSDK()
IAB TCF
Use the code below for an IAB TCF 2.0 Implementation:
private void setOfflineData() throws JSONException {
JSONObject offlineData = new JSONObject();
String otData = readAssetFile(this, "ot_offline_domain_data.json");
if (!TextUtils.isEmpty(otData)) {
offlineData.put("otData", new JSONObject(otData));
}
JSONObject vendorListData = new JSONObject();
String iabData = readAssetFile(this, "ot_offline_iab_data.json");
if (!TextUtils.isEmpty(iabData)) {
vendorListData.put("iabData", new JSONObject(iabData));
}
String googleData = readAssetFile(this, "ot_offline_google_data");
if (!TextUtils.isEmpty(googleData)) {
vendorListData.put("googleData", new JSONObject(googleData));
}
offlineData.put("vendorListData", vendorListData);
new OTPublishersHeadlessSDK().setOTOfflineData(offlineData);
}
// Must call setOfflineData() first before startSDK()
setOfflineData()
startSDK()
Note: Currently setupOTOfflineData() will only work for one JSON at a time, which means we can only support one geolocation rule and one language.
Capture Records of Consent
When a user gives consent, the SDK automatically saves the consent profile locally to disk. This is so a User's settings can be reflected in the Preference Center UI without having to make a network call.
Optionally, a user's consent can be saved to OneTrust servers, this feature is known as Capture Records of Consent and is enabled in the Geolocation Rules section of OneTrust Admin, not on the SDK locally.
Enabling Capture Records of Consent is the only way to feed OneTrust Reports and Dashboards, otherwise the consent data is only kept locally on the device.
Creating Data Subject Profiles
The SDK has the ability to display and manage consent for Universal Consent Purposes, and not just Cookie Categories. For this feature to work, the developer needs to call setShouldCreateProfile(true)
method on the SDKParams
config object.
If this is not done, then the server will only create Consent Receipts instead of also creating Data Subject Profiles when a user updates their choices.
Cross Device Consent
Cross Device Consent is an optional feature. These parameters are not required for initializing the SDK to fetch and display Banners and Preference Centers to users.
That said, if you are enabling the Cross Device Consent functionality, each of these OTProfileSyncParams
are required to be passed to the startSDK()
method to sync user profile data with the latest values on OneTrust servers. More information about the requirements for the JWT that is passed as syncProfileAuth
can be found in the Cross-Device Consent My.OneTrust article.
OTProfileSyncParams.OTProfileSyncParamsBuilder.newInstance()
.setSyncProfile("true")
.setSyncProfileAuth("JWT token")
.setIdentifier("userId")
.setIdentifierType("identifier type");
OTProfileSyncParams otProfileSyncParams = otProfileSyncParamsBuilder.build();
Parameter | Description | Required for Cross Device |
---|---|---|
setSyncProfile | Tells initSDKData to attempt Cross Device Consent profile syncing. | Yes |
setSyncProfileAuth | Use this to pass the pre-signed JWT auth token required to perform Cross Device. | Yes |
setIdentifier | Sets the identifier value used to create a user profile server-side. | Yes |
setIdentifierType | Sets the identifier type for Unified Profile | No, only when Unified Profile is in use |
IMPORTANT
Ensure that you're also setting
shouldCreateProfile("true")
to true inOTSDKParams
so a user profile is created on OneTrust servers.
Cross Device Consent Workflow
By default, when a user logs in and you pass in the cross device consent parameters above, OneTrust will pull down their profile and override the previous anonymous consent (if applicable) if a profile already exists. If a profile doesn't exist, the banner will be resurfaced to collect consent for that profile. For some apps, this can be deemed as an undesirable user experience as you may not want a user to see the banner twice in one instance.
In an effort to improve the user experience above, OneTrust has created functionality that allows the SDK to convert anonymous consent to known consent upon login and not surface the banner again. In the tenant/admin UI, please enable the 'Maintain Consent from Unknown Users After Authentication' toggle. You can find this under Mobile App Consent -> Consent Groups -> Settings. With this toggle/feature enabled, OneTrust will persist the latest consent available upon login. For example, if consent given anonymously (before login) is the latest consent (in terms of the time stamp), then we'll override/update profile consent with anonymous consent even if a profile already exists. If the profile consent time stamp is the latest, then profile consent will override the anonymous consent.
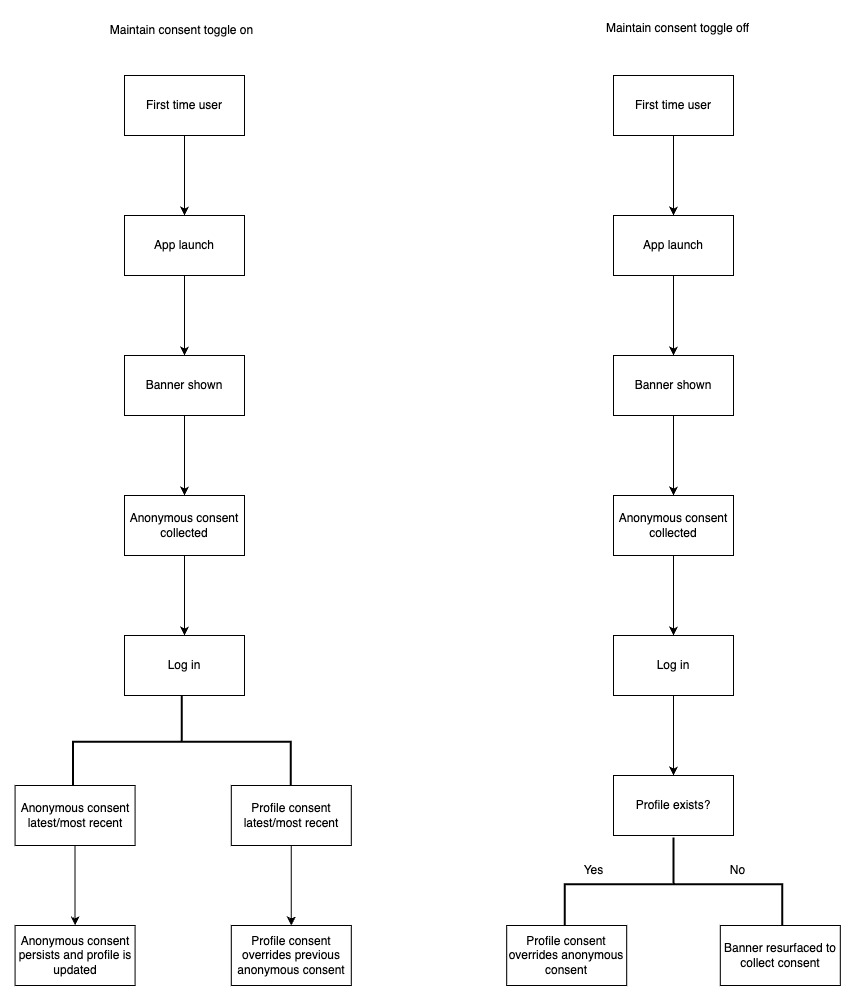
Updated 3 months ago