Display User Interfaces
Overview
The OneTrust SDK manages several different user interfaces to display to a user. Before showing how to display them, please review the list of interfaces below.
IMPORTANT
The SDK renders our UI with AppCompatTheme. If your app uses a theming library and is clashing with ours, reference this section to see how to handle it.
Name | Description | Method to Show |
---|---|---|
Banner | Notice to the user of their privacy rights. Has configurable text and buttons for Accept All, Reject All, Manage Preferences, and Close Banner. Note: Each of these buttons can be toggled On/Off in the Admin Console. | setupUI() showBannerUI() |
Preference Center | An interface for the user to view their current profile settings and update their choices based on the configuration provided for them. Has configurable text and buttons for Accept All, Reject All, Save Settings, and Close Preference Center. Note: You can choose to hide each button except Save Settings - this is required for a User to update their choices. | setupUI() showPreferenceCenterUI() |
Purpose Details | The Purpose Details (or Category Details) view shows granular detail about the category and also shows the SDK LIst link, Vendor List link, and child categories based on configuration. | none, sub-view of Preference Center |
SDK List | An interface to show a granular list of SDKs to the user. This list may be filtered by Category to provide more transparency to the User. Note: This can hidden in Template Settings in the Admin Console. | none, sub-view of Preference Center |
IAB Vendor List | An interface, shown only for IAB2 type templates, displays a list of 3rd party ad tech vendors under management by the application. This provides a way for users to opt in/out of consent for a particular vendor. | none, sub-view of Preference Center |
Vendor Details | A child interface of Vendor List, this view shows more granular information about a vendor and its Purpose, Legitimate Interest, Special Feature, and Special Purpose settings. | none, sub-view of Preference Center |
The user interfaces without methods indicate they can only be navigated to based on User navigation and Admin Portal settings.
setupUI
setupUI
This method can be used to render a UI for Banner or Preference Center. setupUI()
automatically evaluates the shouldShowBanner()
logic to determine whether or not a banner should be shown to users. See below for more info on how shouldShowBanner
gets calculated. See below for more info on how shouldShowBanner() gets calculated.
Param | Description |
---|---|
AppCompatActivity | Reference to the FragmentActivity where the UI will be shown. |
UIType | Either .BANNER , .PREFERENCE_CENTER , .CONSENT_PURPOSES or nil . This determines which UI to display if the shouldShowBanner check is computed to true .a |
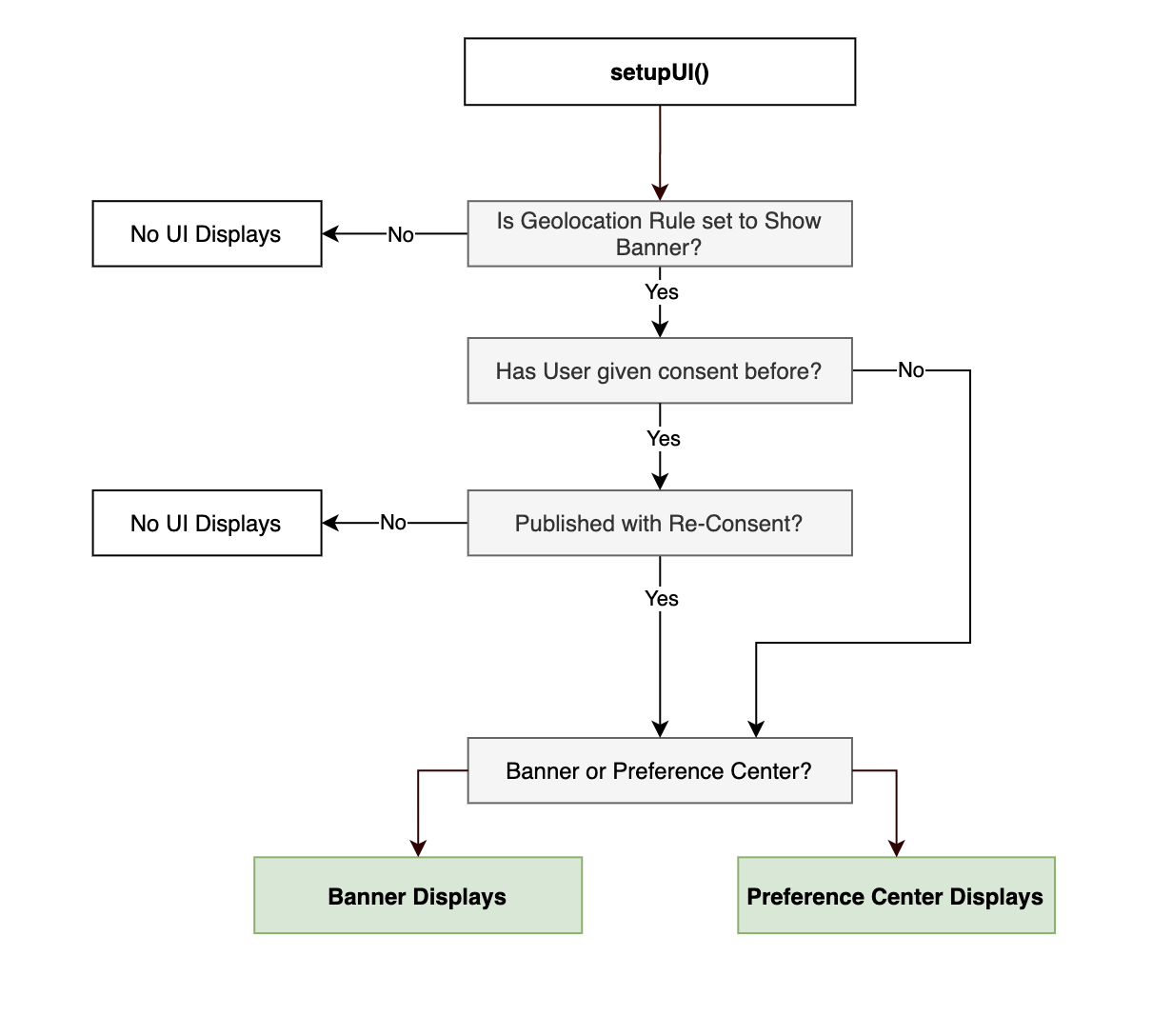
// Java - This will render the OneTrust Mobile Banner and Preference Centers, respectively.
new OTPublishersHeadlessSDK(this).setupUI(RenderNativeUIActivity.this, UIType.BANNER);
new OTPublishersHeadlessSDK(this).setupUI(RenderNativeUIActivity.this, UIType.PREFERENCE_CENTER);
new OTPublishersHeadlessSDK(this).setupUI(RenderNativeUIActivity.this, UIType.CONSENT_PURPOSES);
Recommended Approaches Based on Regulatory Use Case
GDPR / CCPA / CPRA Implementations with Banner
For implementations that require a banner, there are two approaches you can take to surface the banner.
Option 1: shouldShowBanner() + showBannerUI()
This option is recommended for apps that have an involved onboarding process which require you to control when UIs are surfaced from OneTrust.
Example Code:
if shouldShowBanner() {
showBannerUI()
}
else {
//move on to the next step
}
Option 2: setupUI()
This option is recommended for apps that that can show the OneTrust UI without restriction. setupUI()
automatically evaluates shouldShowBanner()
to determine if a banner should be shown.
Example Code:
new OTPublishersHeadlessSDK(this).setupUI(RenderNativeUIActivity.this, UIType.BANNER);
Preference Center
In order to give users the ability to change their consent settings at any time, most implementations require a button (usually behind the settings page) in your app that surfaces the Preference Center. This can be accomplished with showPreferenceCenterUI()
. As this method has no conditional logic attached to it, calling it will surface the preference center every time.
CCPA/CPRA Implementations without Banner
Although no banner is used, most implementations require a button (usually behind the settings page) in your app that surfaces the Preference Center in order to give users the ability to change their consent settings at any time. This can be accomplished with showPreferenceCenterUI()
. As this method has no conditional logic attached to it, calling it will surface the preference center every time.
Example Code:
new OTPublishersHeadlessSDK(this).showPreferenceCenterUI(RenderNativeUIActivity.this);
showBannerUI
showBannerUI
This method will always show the Banner UI, regardless of the shouldShowBanner
value.
Param | Description |
---|---|
AppCompatActivity | Reference to the FragmentActivity where the UI will be be shown. |
new OTPublishersHeadlessSDK(this).showBannerUI(RenderNativeUIActivity.this)
SDK uses activity to get the fragment manager to show the UI.
showPreferenceCenterUI
showPreferenceCenterUI
This method will always show the Preference Center UI, regardless of shouldShowBanner
value.
Param | Description |
---|---|
AppCompatActivity | Reference to the FragmentActivity where the UI will be shown. |
new OTPublishersHeadlessSDK(this).showPreferenceCenterUI(RenderNativeUIActivity.this);
SDK uses activity to get the fragment manager to show the UI.
shouldShowBanner
shouldShowBanner
This method determines if a Banner should be displayed to the user or not based on SDK determined logic.
new OTPublishersHeadlessSDK(this).shouldShowBanner();
How shouldShowBanner
gets computed:
shouldShowBanner
gets computed:-
Is the geolocation rule configured to show a banner? This is controlled by the
showAlertNotice
property in the JSON beingtrue
.- If no, the method returns
false
because a banner is not meant to be shown for this region. - If yes, move to the next check.
- If no, the method returns
-
Is the most recent OT Admin publish configured to re-consent users? This is controlled by the
lastReconsentDate
property in JSON being greater than theLastReconsentDate
last saved on disk.- If yes, the method returns
true
and a banner should be shown. - If no, move to the next check
- If yes, the method returns
-
Is the geolocation rule configured for automatic re-consent AND did the user's automatic re-consent timer expire? This is controlled by measuring the difference between
OneTrustLastConsentDate
saved on disk and theCurrent Date
and seeing if it is greater than or equal toOneTrustReconsentFrequencyDays
in the JSON saved on disk. The SDK considers the date itself and not the time consent was given. For example, if the re-consent period is set to 1 day in my geolocation rule and the user provides consent at 11:59pm GMT, the banner will reappear at 12:00am GMT (1 minute later, but the next day).- If yes, the method returns
true
and a banner should be shown. - If no, move to the next check
- If yes, the method returns
-
Is the Cross Device Consent feature enabled and were 100% of Purposes synced? This is controlled by the
profile.sync.allPurposesUpdatedAfterSync
property in JSON beingtrue
.- If yes, the method returns
false
and a banner should not be shown. This is because each of the Categories/Purposes managed by the SDK were 100% synced from the user's profile on the OneTrust server. - If no, move to the next check.
- If yes, the method returns
-
Has the user previously given consent?
- If yes, the method returns
false
and a banner should not be shown. - If no, the method returns
true
and a banner should be shown because this is the first time they are being asked to give consent on the app.
- If yes, the method returns
-
Has a new category been added post giving consent?
- If yes, the method returns
true
and a banner should be shown again because user needs to give consent to the new category. - If no, the method returns
false
and a banner should not be shown.
- If yes, the method returns
Note: If you are using OneTrust's pre-built UI, it is likely you won't need to call this method, but good to know how it works as it is decided the outcome of
setupUI
method.Note: If you are building your own UI (BYOUI), then the method below will likely be part of your implementation.
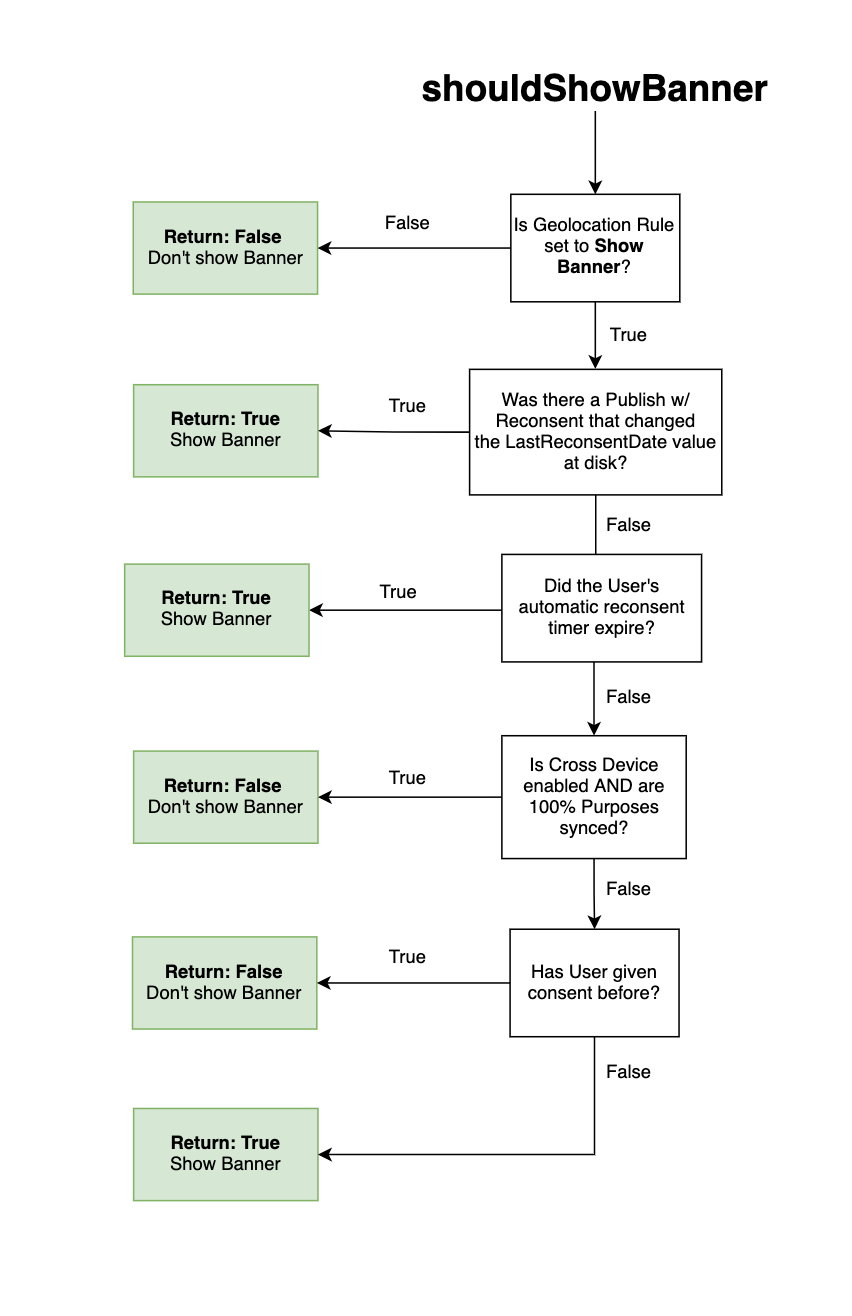
isBannerShown
isBannerShown
This method is used, in some implementations, where the application needs to hide a "Privacy Settings" application tab or prevent a user from accessing the Preference Center before they have seen the Banner notice at least once.
The logic for computing this value is:
- Returns
-1
when the SDK has not been initialized yet - Returns
0
when a Banner / Preference Center has never been shown - Returns
1
when a Banner / Preference Center has been shown - Returns
2
when the Banner / Preference Center has never been shown, but the User Profile was synced automatically (only applicable for implementations using Cross Device profile syncing).
For most implementations, the application logic can be:
- If the value returned is greater than 0, then show "Privacy Settings" or some link to show a Preference Center.
new OTPublishersHeadlessSDK(this).isBannerShown()
showConsentUI
showConsentUI
This method surfaces prompts for gathering different types of consent such as an age gate.
Param | Description |
---|---|
AppCompatActivity | Reference to the FragmentActivity where the UI will be be shown. |
UIType | Pass .AGE_GATE in order to render the Age Gate UI. |
new OTPublishersHeadlessSDK(this).showConsentUI(RenderNativeUIActivity.this, PromptUIType.AGE_GATE, otConfiguration, new OTConsentUICallback() {
@Override
public void onCompletion() {
}
});
Note: The below referenced UIType param can be used to integrate your Universal Consent Preferences into the Mobile Preference Center interface. This feature set is behind a feature toggle. Please contact your OneTrust implementation consultant for access. Reference this MyOneTrust article for configuration guidance.
setOTSDKTheme
setOTSDKTheme
Some apps use popular theming libraries like Material which can sometimes cause issues with some of the OneTrust UI styles.
If this situation occurs, your application can force OneTrust to load all generated interfaces using the AppCompat theme. This setting is passed to the the startSDK
call.
Note: The SDK does not support Material theme with this approach, this is more of a safeguard. The OneTrust team will be exploring better ways to support Android theming libraries like Material in future releases.
OTUXParams uxParams = OTUXParams.OTUXParamsBuilder.newInstance()
.setOTSDKTheme(OTThemeConstants.OT_THEME_APP_COMPACT_LIGHT_NO_ACTION_BAR)
.build();
OTSdkParams sdkParams = OTSdkParams.SdkParamsBuilder.newInstance()
.setOTUXParams(uxParams)
.build();
otPublishersHeadlessSDK.startSDK(myApp.storage, myApp.appId, "en", sdkParams, myCallback}
If you're still seeing issues with the UI after setting the method above, we recommend setting @style/AppTheme
in the Manifest file where you're rendering the activity. If this is not possible, the following workaround can be explored:
Theming Library Workaround
Create a separate AppCompatActivity that is presented on top of your MainActivity. This should prevent you from having to change the theme of your app.
- Create a new Activity in your AndroidManifest.xml that uses the AppTheme. We'll call it “TemporaryBanner”.
<activity
android:name=".TemporaryBanner"
android:theme="@style/Theme.AppCompat.NoActionBar"
android:exported="false" />
</activity>
- Create a new file which we'll call TemporaryBanner.java. Instantiate OTPublishersHeadlessSDK and call your UI method.
public class TemporaryBanner extends AppCompatActivity {
OTPublishersHeadlessSDK ot;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_temporary_banner);
ot = new OTPublishersHeadlessSDK(this);
ot.showBannerUI(this);
setEventListeners();
}
}
- Create a new method in your MainActivity that will start the UI in a new Activity.
private void showBannerOnNewActivity() {
Intent intent = new Intent(this.getApplicationContext(), TemporaryBanner.class);
this.startActivity(intent);
}
- Call showBannerOnNewActivity() in the onSuccess handler of startSDK() or wherever you'd like to call it.
public void onSuccess(@NonNull OTResponse otResponse) {
...
showBannerOnNewActivity()
}
- Set OTEventListeners in TemporaryBanner.java so that we end the activity on allSDKsViewDismissed() after the user has either dismissed the banner or preference center.
@Override
public void allSDKViewsDismissed(String s) {
TemporaryBanner.super.finish();
}
Edge-to-Edge
If your app uses styles like Edge-to-Edge that causes discrepancies with the OneTrust UI, you can set an additional theme to correct the issue.
OTUXParams uxParams = OTUXParams.OTUXParamsBuilder.newInstance()
.setOTSDKTheme(OTThemeConstants.OT_SDK_UI_THEME)
.build();
OTSdkParams sdkParams = OTSdkParams.SdkParamsBuilder.newInstance()
.setOTUXParams(uxParams)
.build();
otPublishersHeadlessSDK.startSDK(myApp.storage, myApp.appId, "en", sdkParams, myCallback}
dismissUI
dismissUI
This method can be used to dismiss the OneTrust SDK's UI at any time. Although dismissing the OneTrust UI is often handled by a user saving their choices, there are some situations where the application may want manual control over this:
When this method is called:
- The Banner/PC UI will be dismissed from view
- Consent will NOT be saved or logged to OneTrust
- Any Category/Purpose value updates will be Reset
- The value of
shouldShowBanner
will remain Same - A warning message will be logged if this method is called and OneTrust UI is not currently being shown
Param | Description |
---|---|
FragmentActivity | Reference to the FragmentActivity where the OT SDK's UI is currently being shown. |
new OTPublishersHeadlessSDK(this).dismissUI(MainActivity.this);
showConsentPurposesUI
showConsentPurposesUI
This method displays the Universal Consent Preference Center. Universal Consent is a separate module and serves a different use case than Mobile App Consent. For more information, reference the following document: https://my.onetrust.com/s/article/UUID-23e87737-2b1d-f741-5ab3-400d06c4bdd4
// java
new OTPublishersHeadlessSDK(this).showConsentPurposesUI(RenderNativeUIActivity.this)
shouldEnableDarkMode
shouldEnableDarkMode
This method will enable Dark Mode and can override existing Dark Mode configurations on a device.
To enable dark mode, pass param as "true"
.
// java
OTConfiguration otConfiguration =
OTConfiguration.OTConfigurationBuilder.newInstance().shouldEnableDarkMode("true").build();
otPublishersHeadlessSDK.showBannerUI(RenderNativeUIActivity.this,otConfiguration);
Updated 2 months ago